Java FAQ
Frequently Asked Questions
This page provides a reference to the Java programming language as covered in this course.
It is not meant to be a complete reference.
For that refer to the on-line documentation and to the Sun web site.
Click on one of the questions below to go to the ansewer.
Click on the Back button on the tool bar to return to the list of questions.
-
How does Java work?
-
What makes up a Java program?
-
What does all that stuff in the HelloWorld program mean?
-
What are the 10 most common programming errors in Java?
How does Java work?
Using a text editor you write your Source Code and save it with the .java
extension. This is then translated by a special program called a Compiler into
Java Byte Code in a file with the .class extension. This program is then
run by the Java Runtime Engine (JRE) which has been installed on your computer.
Java can run on a Windows PC, a Macintosh, a Linux PC, a Sun workstation, or any number
of other machines.
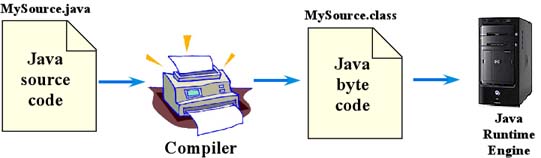
Java Code: From source to byte code to executing program.
What makes up a Java program?
The source code for a Java program consists of one or more packages usually
named with the prefix pkg such as pkgMyPackage. Some small programs
created in Eclipse may just use the (default package). Each package contains
one or more Source Code files,
each with the .java extension. Each source code file defines a block of
code defining a Class. Just inside this class (below the opening brace '{')
will be defined a number of Member Variables. These are variables which can
be accessed and used anywhere within the program. The class will also contain a
Constructor function. This function gets automatically called whenever a
running Java program creates a new instance of the class. This is where everything is
initialized. In addition to the constructor the class may contain a number of Methods
each of which contains a block of code designed to so something in the program. Methods
are also known as Functions. Each method or function contains a number of
Statements. Statements are the actual commands which tell the computer what
to do. In one and only one source code file will be found a special method called
main. This is where a Java program actually begins when you run it.
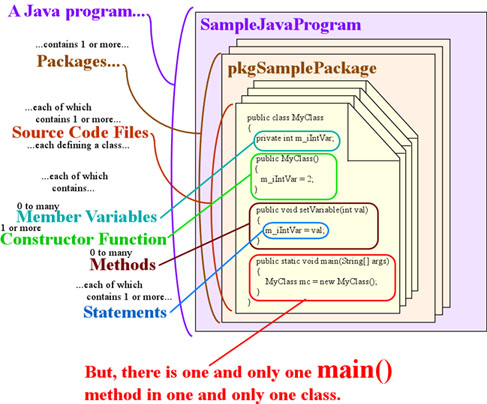
Parts of a Java Program
What does all that mean in the HelloWorld program?
For many years it has been a tradition among programmers that when learning a new
language your first program should just print Hello, World! In this course,
following that tradition, the first Java program does just that. The source code
for that program is listed below:
/** This is a very simple Java application.
* It prints the traditional "Hello World" message.
*/
public class HelloWorld
{
// main(). The starting point of all programs
public static void main(String[] args)
{
// Print the message
System.out.println("Hello world.");
}
}
-
Comments - You can place comments anywhere in your code to help
you remember what you were doing at that point. The compiler ignores any
comment lines. You can create a multi-line comment by starting it with /*
(slash-asterisk) or /** (slash-asterisk-asterisk) and ending it with
*/ (asterisk-slash). Be careful in Eclipse because if you
start a comment this way the first time you press the Enter key to move to a
new line it will automatically add the closing */ for you. You can also create
a comment by starting it with // (slash-slash). This comment ends at the
end of the line. You see both kinds of comments in the HelloWorld program. To make
comments easy to see, Eclipse colors their text green.
-
public – This is called an Access Specifier. It determines who or what can
access a thing (class, method, variable). Public means everyone and everything
can access this class. There are two other access specifiers: private and
protected. As the names imply these restrict who or what can access the things.
-
class – This is a special type of object. Think of a class as a building
block of Java programs. The HelloWorld program consists of only one class. Most Java
program consist of many classes.
-
HelloWorld – This is the name of the class.
-
{ – Opening curly brace. This is the start of the class definition body. All blocks
of code in a Java program must be enclosed in braces. When you see code printed or displayed
on the screen be careful to not get braces mixed up with parentheses. Eclipse will give you
an error message if you do, but sometimes the message is not very clear.
-
public – Again, so everyone can get to it. Methods and classes both must have an
access specifier.
-
static – This is a special type of method. A beginning programmer doesn't need to
have their brain muddled with why this is there, just remember that it must always be include
in this spot for the main method.
-
void – All methods return a value of some type unless the return type is designated as
void. This just means there is no return value, i.e. the return value is empty or "void".
-
main – This is the name of this method. For most methods you can give them any name that
makes sense to you, but this method must always be called main. When you run your Java
program it always starts in main.
-
(String[] args) – When a method is "called", meaning program execution is transferred to
the block of code in the method you can pass to the method any data that would be needed for the
method to do its' job. This data is referred to as arguments to the method. They are
enclosed in parentheses. In this case it means main() gets and array (indicated by the []
brackets) of type String (words or text) and the array is named, appropriately enough,
args. Actually you can name the arguments to a method anything you want, but in this case
the name just seems to make sense.
-
{ – The opening curly brace. The start of the method definition body.
-
System.out.println – This tells the Java System object to use a special set of
methods referred to as the out (for output) object, and specifically to use the
println (print line) method to print to the standard output, i.e. the command line
on the screen.
-
("Hello world.") – This is the argument to the println method which is the
string, enclosed in quotes, that you want to print. As with all calls to methods the
arguments are enclosed in parentheses after the name of the method being called.
-
; – Every statement in a Java program must end with a semicolon. If you forget the
semicolon Eclipse will give you an error message, but it may not be exactly clear as to what
the error is. Leaving out semicolons can sometime confuse the compiler, just like a "run-on
sentence" can confuse you when trying to read it. Note that you do not put a semicolon at
the end of a method header or class header. That is because those are only the starting of
the following block of code which you enclose in curly braces.
-
} – The closing curly brace which marks the end of the main method.
-
} – The closing curly brace which marks the end of the class definition.
What some of the 10 most common programming errors in Java?
-
Misspelled words -- Java is case sensitive. If Eclipse flags an error check your spelling.
-
Failure to add a needed import -- Everything in the Java Class Library which is to be used
in a class must be added to the class via an import statement.
-
Unbalanced {([ or ])} -- Every opening brace, parenthesis, and bracket must have a closing one.
-
Misplaced {([ or ])} -- Braces, parentheses, and brackets are used to enclose blocks of code,
argument lists, array indices, etc. They must be in the correct order.
-
Misplaced code -- All code must be inside of functions. Code placed outside of functions, such
as where class member variables are defined will be flagged as errors even if it
is syntactically correct.
-
Incorrect or lack of use of () in math functions -- In the statement int X = 10 - 8 / 4 - 2;
you may want to divide 10-8 by 4-2, but X will be set to 6 (* and / is done before + and - ). To get the desired
answer use X=(10-8)/(4-2);
-
Incorrect function names -- Make sure the function name is correct, i.e. setBackground not setBackgroundColor.
-
Incorrect punctuation operators -- Make sure the correct punctuation operator is used, i.e. : ; , .
-
Omitting the semicolon at the end of a statement. -- All statements must end with a semicolon (;)
to mark the end of the statement.
-
Misplaced semicolons -- Semicolons mark the end of statements, so they cannot be placed at the end of the
opening of a for or while loop.

This site is still under construction