Demonstrations of Java Layout Managers
Java provides five layout managers for handling the layout of components in a GUI.
This can be most useful if the window the GUI widgets are contained in is resized.
The code shown below will create a single window (Figure 1) with a number of buttons
on it. This window was created with null layout manager, meaning that the
size and location of all the buttons was defined in code. The window was also set
so it cannot be resized. Clicking a button will bring up a sample window demonstrating
a layout manager with five buttons placed in a panel. For more information check
the Java Docs site .
This project was created in Eclipse. It is assumed you are familiar with Eclipse and
know how to create projects, and add packages and classes to a project.
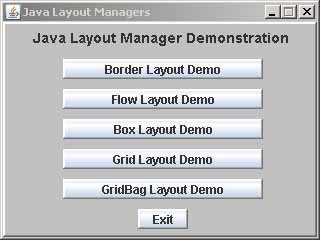
Figure 1: Main window
-
Border Layout Manager
This is the default layout manager. Items are placed at the positions:
NORTH, SOUTH, EAST, WEST and CENTER.
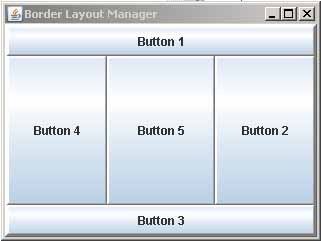
Figure 2: BorderLayout
-
Flow Layout Manager
The Flow layout manager places items all on one row from left to
right. If there is not enough room on one row then multiple rows are
used. Items on each row are centered in the frame.
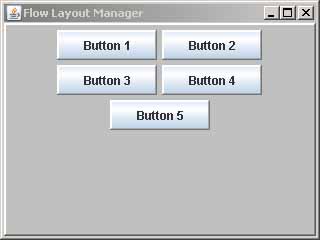
Figure 2: BorderLayout
-
Box Layout Manager
The Box layout manager places items based on a set orientation pattern.
This pattern can be: X_AXIS - components are laid out in one row, left to
right; Y_AXIS - components are laid out in one column, top to bottom;
LINE_AXIS - components are laid out like words in a line, i.e. left to
right and in multiple rows as needed (like the Flow layout manager.);
PAGE_AXIS - components are laid out vertically, top to bottom and in
multiple columns, if needed, from left to right.
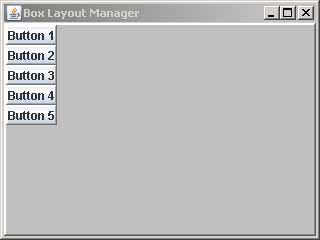
Figure 2: BorderLayout
-
Grid Layout Manager
The Grid layout manager places items in rows (left to right) and columns
(top to bottom). The number of rows and columns is defined when the Grid
layout manager is created.
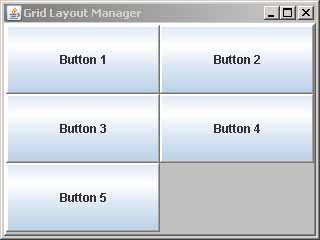
Figure 2: BorderLayout
-
GridBag Layout Manager
The GridBag layout manager is, by far, the most complicated to use and the
details are too involved to cover here. For more information check out the
Java Doc link above, the
Java Tutorial and the comments in the code demo below.
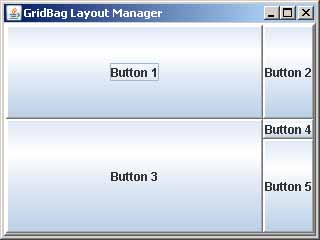
Figure 2: BorderLayout
Source listing for the Layout Manager Demonstration
package pkgLayoutManagerDemo;
import java.awt.Color;
import java.awt.Font;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.BorderFactory;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.border.BevelBorder;
//=============================================================================
/** This program demonstrates each of the Java Layout Managers. For each
* demo a JFrame containing a JPanel is created and 5 JButtons are added
* to the JPanel.
* @author Dr. Rick Coleman
* Date: February 2009
*/
//=============================================================================
public class LayoutManagerDemo extends JFrame
{
/** Main panel for the demonstration */
private JPanel m_MainPanel;
/** Show the BorderLayout demo button */
private JButton m_BorderBtn;
/** Show the FlowLayout demo button*/
private JButton m_FlowBtn;
/** Show the BoxLayout demo button*/
private JButton m_BoxBtn;
/** Show the GridLayout demo button*/
private JButton m_GridBtn;
/** Show the GridBagLayout demo button*/
private JButton m_GridBagBtn;
/** Exit button*/
private JButton m_ExitBtn;
/** Show the BorderLayout demo window */
private BorderDemo m_BorderDemo;
/** Show the FlowLayout demo window */
private FlowDemo m_FlowDemo;
/** Show the BoxLayout demo window */
private BoxDemo m_BoxDemo;
/** Show the GridLayout demo window */
private GridDemo m_GridDemo;
/** Show the GridBagLayout demo window */
private GridBagDemo m_GridBagDemo;
/** Label font used for most labels */
public static final Font SysLabelFontB = new Font("SansSerif", Font.BOLD, 14);
//------------------------------------------------------------
/** Default constructor */
//------------------------------------------------------------
public LayoutManagerDemo()
{
this.setSize(320, 240);
this.setLocation(425, 100);
this.setBackground(Color.LIGHT_GRAY);
this.setDefaultCloseOperation(EXIT_ON_CLOSE);
this.setTitle("Java Layout Managers");
this.setResizable(false);
// Create the main panel
m_MainPanel = new JPanel();
m_MainPanel.setSize(320, 400);
m_MainPanel.setLocation(0, 0);
m_MainPanel.setLayout(null);
m_MainPanel.setBackground(Color.LIGHT_GRAY);
m_MainPanel.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
this.getContentPane().add(m_MainPanel);
// Create a general use label
JLabel tempLbl;
tempLbl = new JLabel("Java Layout Manager Demonstration");
tempLbl.setSize(300, 20);
tempLbl.setFont(SysLabelFontB);
tempLbl.setLocation(30, 5);
m_MainPanel.add(tempLbl);
int fudge = -3;
// Create and add the Border demo button
m_BorderBtn = new JButton("Border Layout Demo");
m_BorderBtn.setSize(200, 20);
m_BorderBtn.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
m_BorderBtn.setLocation(60, 40 + fudge);
m_BorderBtn.addActionListener(
new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
// Show the Border demo
m_BorderDemo.setVisible(true);
}
});
m_MainPanel.add(m_BorderBtn);
// Create and add the Flow demo button
m_FlowBtn = new JButton("Flow Layout Demo");
m_FlowBtn.setSize(200, 20);
m_FlowBtn.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
m_FlowBtn.setLocation(60, 70 + fudge);
m_FlowBtn.addActionListener(
new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
// Show the Flow demo
m_FlowDemo.setVisible(true);
}
});
m_MainPanel.add(m_FlowBtn);
// Create and add the Box demo button
m_BoxBtn = new JButton("Box Layout Demo");
m_BoxBtn.setSize(200, 20);
m_BoxBtn.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
m_BoxBtn.setLocation(60, 100 + fudge);
m_BoxBtn.addActionListener(
new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
// Show the Box demo
m_BoxDemo.setVisible(true);
}
});
m_MainPanel.add(m_BoxBtn);
// Create and add the Grid demo button
m_GridBtn = new JButton("Grid Layout Demo");
m_GridBtn.setSize(200, 20);
m_GridBtn.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
m_GridBtn.setLocation(60, 130 + fudge);
m_GridBtn.addActionListener(
new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
// Show the Grid demo
m_GridDemo.setVisible(true);
}
});
m_MainPanel.add(m_GridBtn);
// Create and add the GridBag demo button
m_GridBagBtn = new JButton("GridBag Layout Demo");
m_GridBagBtn.setSize(200, 20);
m_GridBagBtn.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
m_GridBagBtn.setLocation(60, 160 + fudge);
m_GridBagBtn.addActionListener(
new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
// Show the GridBag demo
m_GridBagDemo.setVisible(true);
}
});
m_MainPanel.add(m_GridBagBtn);
// Create and add the Exit button
m_ExitBtn = new JButton("Exit");
m_ExitBtn.setSize(50, 20);
m_ExitBtn.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
m_ExitBtn.setLocation(135, 190 + fudge);
m_ExitBtn.addActionListener(
new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
System.exit(0);
}
});
m_MainPanel.add(m_ExitBtn);
// Create all the demo frames
m_BorderDemo = new BorderDemo();
m_BorderDemo.setVisible(false);
m_FlowDemo = new FlowDemo();
m_FlowDemo.setVisible(false);
m_BoxDemo = new BoxDemo();
m_BoxDemo.setVisible(false);
m_GridDemo = new GridDemo();
m_GridDemo.setVisible(false);
m_GridBagDemo = new GridBagDemo();
m_GridBagDemo.setVisible(false);
// Show the demo window
this.setVisible(true);
}
public static void main(String[] args)
{
LayoutManagerDemo lmd = new LayoutManagerDemo();
}
}
Source listing for the Border Layout Manager Demonstration
package pkgLayoutManagerDemo;
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.BorderFactory;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.BevelBorder;
//=============================================================================
/** This class demonstrates the Java Border Layout Manager.
* @author Dr. Rick Coleman
* Date: February 2009
*/
//=============================================================================
public class BorderDemo extends JFrame
{
/** Main panel for the demonstration */
private JPanel m_MainPanel;
/** Button 1 */
private JButton m_Button1;
/** Button 2 */
private JButton m_Button2;
/** Button 3 */
private JButton m_Button3;
/** Button 4 */
private JButton m_Button4;
/** Button 5 */
private JButton m_Button5;
//----------------------------------------------------------
/** Default constructor */
//----------------------------------------------------------
public BorderDemo()
{
this.setSize(320, 240);
this.setLocation(750, 150);
this.setBackground(Color.LIGHT_GRAY);
this.setDefaultCloseOperation(EXIT_ON_CLOSE);
this.setTitle("Border Layout Manager");
this.setResizable(true);
// Create the main panel
m_MainPanel = new JPanel();
m_MainPanel.setSize(320, 220);
m_MainPanel.setLocation(0, 0);
m_MainPanel.setLayout(new BorderLayout());
m_MainPanel.setBackground(Color.LIGHT_GRAY);
m_MainPanel.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
this.getContentPane().add(m_MainPanel);
// Create and add the button 1
m_Button1 = new JButton("Button 1");
m_Button1.setPreferredSize(new Dimension(100, 30));
m_Button1.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
m_Button1.addActionListener(
new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
}
});
m_MainPanel.add(BorderLayout.NORTH, m_Button1);
// Create and add the button 2
m_Button2 = new JButton("Button 2");
m_Button2.setPreferredSize(new Dimension(100, 30));
m_Button2.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
m_Button2.addActionListener(
new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
}
});
m_MainPanel.add(BorderLayout.EAST, m_Button2);
// Create and add the button 3
m_Button3 = new JButton("Button 3");
m_Button3.setPreferredSize(new Dimension(100, 30));
m_Button3.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
m_Button3.addActionListener(
new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
}
});
m_MainPanel.add(BorderLayout.SOUTH, m_Button3);
// Create and add the button 4
m_Button4 = new JButton("Button 4");
m_Button4.setPreferredSize(new Dimension(100, 30));
m_Button4.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
m_Button4.addActionListener(
new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
}
});
m_MainPanel.add(BorderLayout.WEST, m_Button4);
// Create and add the button 5
m_Button5 = new JButton("Button 5");
m_Button5.setPreferredSize(new Dimension(100, 30));
m_Button5.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
m_Button5.addActionListener(
new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
}
});
m_MainPanel.add(BorderLayout.CENTER, m_Button5);
}
}
Source listing for the Flow Layout Manager Demonstration
package pkgLayoutManagerDemo;
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.FlowLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.BorderFactory;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.BevelBorder;
//=============================================================================
/** This class demonstrates the Java Flow Layout Manager.
* @author Dr. Rick Coleman
* Date: February 2009
*/
//=============================================================================
public class FlowDemo extends JFrame
{
/** Main panel for the demonstration */
private JPanel m_MainPanel;
/** Button 1 */
private JButton m_Button1;
/** Button 2 */
private JButton m_Button2;
/** Button 3 */
private JButton m_Button3;
/** Button 4 */
private JButton m_Button4;
/** Button 5 */
private JButton m_Button5;
//----------------------------------------------------------
/** Default constructor */
//----------------------------------------------------------
public FlowDemo()
{
this.setSize(320, 240);
this.setLocation(100, 150);
this.setBackground(Color.LIGHT_GRAY);
this.setDefaultCloseOperation(EXIT_ON_CLOSE);
this.setTitle("Flow Layout Manager");
this.setResizable(true);
// Create the main panel
m_MainPanel = new JPanel();
m_MainPanel.setSize(320, 220);
m_MainPanel.setLocation(0, 0);
m_MainPanel.setLayout(new FlowLayout());
m_MainPanel.setBackground(Color.LIGHT_GRAY);
m_MainPanel.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
this.getContentPane().add(m_MainPanel);
// Create and add the button 1
m_Button1 = new JButton("Button 1");
m_Button1.setPreferredSize(new Dimension(100, 30));
m_Button1.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
m_Button1.addActionListener(
new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
}
});
m_MainPanel.add(m_Button1);
// Create and add the button 2
m_Button2 = new JButton("Button 2");
m_Button2.setPreferredSize(new Dimension(100, 30));
m_Button2.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
m_Button2.addActionListener(
new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
}
});
m_MainPanel.add(m_Button2);
// Create and add the button 3
m_Button3 = new JButton("Button 3");
m_Button3.setPreferredSize(new Dimension(100, 30));
m_Button3.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
m_Button3.addActionListener(
new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
}
});
m_MainPanel.add(m_Button3);
// Create and add the button 4
m_Button4 = new JButton("Button 4");
m_Button4.setPreferredSize(new Dimension(100, 30));
m_Button4.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
m_Button4.addActionListener(
new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
}
});
m_MainPanel.add(m_Button4);
// Create and add the button 5
m_Button5 = new JButton("Button 5");
m_Button5.setPreferredSize(new Dimension(100, 30));
m_Button5.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
m_Button5.addActionListener(
new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
}
});
m_MainPanel.add(m_Button5);
}
}
Source listing for the Box Layout Manager Demonstration
package pkgLayoutManagerDemo;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.FlowLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.BorderFactory;
import javax.swing.BoxLayout;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.BevelBorder;
//=============================================================================
/** This class demonstrates the Java Box Layout Manager.
* @author Dr. Rick Coleman
* Date: February 2009
*/
//=============================================================================
public class BoxDemo extends JFrame
{
/** Main panel for the demonstration */
private JPanel m_MainPanel;
/** Button 1 */
private JButton m_Button1;
/** Button 2 */
private JButton m_Button2;
/** Button 3 */
private JButton m_Button3;
/** Button 4 */
private JButton m_Button4;
/** Button 5 */
private JButton m_Button5;
//----------------------------------------------------------
/** Default constructor */
//----------------------------------------------------------
public BoxDemo()
{
this.setSize(320, 240);
this.setLocation(750, 450);
this.setBackground(Color.LIGHT_GRAY);
this.setDefaultCloseOperation(EXIT_ON_CLOSE);
this.setTitle("Box Layout Manager");
this.setResizable(true);
// Create the main panel
m_MainPanel = new JPanel();
m_MainPanel.setSize(320, 220);
m_MainPanel.setLocation(0, 0);
m_MainPanel.setLayout(new BoxLayout(m_MainPanel, BoxLayout.PAGE_AXIS));
m_MainPanel.setBackground(Color.LIGHT_GRAY);
m_MainPanel.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
this.getContentPane().add(m_MainPanel);
// Create and add the button 1
m_Button1 = new JButton("Button 1");
m_Button1.setPreferredSize(new Dimension(100, 30));
m_Button1.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
m_Button1.addActionListener(
new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
}
});
m_MainPanel.add(m_Button1);
// Create and add the button 2
m_Button2 = new JButton("Button 2");
m_Button2.setPreferredSize(new Dimension(100, 30));
m_Button2.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
m_Button2.addActionListener(
new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
}
});
m_MainPanel.add(m_Button2);
// Create and add the button 3
m_Button3 = new JButton("Button 3");
m_Button3.setPreferredSize(new Dimension(100, 30));
m_Button3.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
m_Button3.addActionListener(
new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
}
});
m_MainPanel.add(m_Button3);
// Create and add the button 4
m_Button4 = new JButton("Button 4");
m_Button4.setPreferredSize(new Dimension(100, 30));
m_Button4.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
m_Button4.addActionListener(
new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
}
});
m_MainPanel.add(m_Button4);
// Create and add the button 5
m_Button5 = new JButton("Button 5");
m_Button5.setPreferredSize(new Dimension(100, 30));
m_Button5.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
m_Button5.addActionListener(
new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
}
});
m_MainPanel.add(m_Button5);
}
}
Source listing for the Grid Layout Manager Demonstration
package pkgLayoutManagerDemo;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.FlowLayout;
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.BorderFactory;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.BevelBorder;
//=============================================================================
/** This class demonstrates the Java Grid Layout Manager.
* @author Dr. Rick Coleman
* Date: February 2009
*/
//=============================================================================
public class GridDemo extends JFrame
{
/** Main panel for the demonstration */
private JPanel m_MainPanel;
/** Button 1 */
private JButton m_Button1;
/** Button 2 */
private JButton m_Button2;
/** Button 3 */
private JButton m_Button3;
/** Button 4 */
private JButton m_Button4;
/** Button 5 */
private JButton m_Button5;
//----------------------------------------------------------
/** Default constructor */
//----------------------------------------------------------
public GridDemo()
{
this.setSize(320, 240);
this.setLocation(100, 450);
this.setBackground(Color.LIGHT_GRAY);
this.setDefaultCloseOperation(EXIT_ON_CLOSE);
this.setTitle("Grid Layout Manager");
this.setResizable(true);
// Create the main panel
m_MainPanel = new JPanel();
m_MainPanel.setSize(320, 220);
m_MainPanel.setLocation(0, 0);
m_MainPanel.setLayout(new GridLayout(0, 2)); // 2 columns any number of rows
m_MainPanel.setBackground(Color.LIGHT_GRAY);
m_MainPanel.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
this.getContentPane().add(m_MainPanel);
// Create and add the button 1
m_Button1 = new JButton("Button 1");
m_Button1.setPreferredSize(new Dimension(100, 30));
m_Button1.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
m_Button1.addActionListener(
new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
}
});
m_MainPanel.add(m_Button1);
// Create and add the button 2
m_Button2 = new JButton("Button 2");
m_Button2.setPreferredSize(new Dimension(100, 30));
m_Button2.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
m_Button2.addActionListener(
new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
}
});
m_MainPanel.add(m_Button2);
// Create and add the button 3
m_Button3 = new JButton("Button 3");
m_Button3.setPreferredSize(new Dimension(100, 30));
m_Button3.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
m_Button3.addActionListener(
new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
}
});
m_MainPanel.add(m_Button3);
// Create and add the button 4
m_Button4 = new JButton("Button 4");
m_Button4.setPreferredSize(new Dimension(100, 30));
m_Button4.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
m_Button4.addActionListener(
new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
}
});
m_MainPanel.add(m_Button4);
// Create and add the button 5
m_Button5 = new JButton("Button 5");
m_Button5.setPreferredSize(new Dimension(100, 30));
m_Button5.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
m_Button5.addActionListener(
new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
}
});
m_MainPanel.add(m_Button5);
}
}
Source listing for the GridBag Layout Manager Demonstration
package pkgLayoutManagerDemo;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.GridBagConstraints;
import java.awt.GridBagLayout;
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.BorderFactory;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.border.BevelBorder;
//=============================================================================
/** This class demonstrates the Java GridBag Layout Manager.
* @author Dr. Rick Coleman
* Date: February 2009
*/
//=============================================================================
public class GridBagDemo extends JFrame
{
/** Main panel for the demonstration */
private JPanel m_MainPanel;
/** Button 1 */
private JButton m_Button1;
/** Button 2 */
private JButton m_Button2;
/** Button 3 */
private JButton m_Button3;
/** Button 4 */
private JButton m_Button4;
/** Button 5 */
private JButton m_Button5;
//----------------------------------------------------------
/** Default constructor */
//----------------------------------------------------------
public GridBagDemo()
{
this.setSize(320, 240);
this.setLocation(425, 550);
this.setBackground(Color.LIGHT_GRAY);
this.setDefaultCloseOperation(EXIT_ON_CLOSE);
this.setTitle("GridBag Layout Manager");
this.setResizable(true);
// Create the main panel
m_MainPanel = new JPanel();
m_MainPanel.setSize(320, 220);
m_MainPanel.setLocation(0, 0);
GridBagLayout gbl = new GridBagLayout(); // Create the manager
m_MainPanel.setLayout(gbl);
m_MainPanel.setBackground(Color.LIGHT_GRAY);
m_MainPanel.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
this.getContentPane().add(m_MainPanel);
// Create a GridBagConstraints to set everything
GridBagConstraints gbc = new GridBagConstraints();
gbc.fill = GridBagConstraints.BOTH;
// Create and add the button 1
m_Button1 = new JButton("Button 1");
m_Button1.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
m_Button1.addActionListener(
new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
}
});
// Set the constraints
gbc.weightx = 1.0;
gbc.weighty = 1.0;
gbc.gridx = 0;
gbc.gridy = 0;
gbc.gridwidth = 1;
gbc.gridheight = 1;
gbl.setConstraints(m_Button1, gbc);
m_MainPanel.add(m_Button1);
// Create and add the button 2
m_Button2 = new JButton("Button 2");
m_Button2.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
m_Button2.addActionListener(
new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
}
});
// Set the constraints
gbc.weightx = 0.0; // Reset to default
gbc.gridx = GridBagConstraints.RELATIVE; // Reset to default
gbc.gridy = GridBagConstraints.RELATIVE; // Reset to default
gbc.gridwidth = GridBagConstraints.REMAINDER;
gbl.setConstraints(m_Button2, gbc);
m_MainPanel.add(m_Button2);
// Create and add the button 3
m_Button3 = new JButton("Button 3");
m_Button3.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
m_Button3.addActionListener(
new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
}
});
// Set the constraints
gbc.gridwidth = 1; //reset to the default
gbc.gridheight = 2;
gbc.weighty = 1.0;
gbl.setConstraints(m_Button3, gbc);
m_MainPanel.add(m_Button3);
// Create and add the button 4
m_Button4 = new JButton("Button 4");
m_Button4.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
m_Button4.addActionListener(
new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
}
});
// Set the constraints
gbc.weighty = 0.0; //reset to the default
gbc.gridwidth = GridBagConstraints.REMAINDER; //end row
gbc.gridheight = 1; //reset to the default
gbl.setConstraints(m_Button4, gbc);
m_MainPanel.add(m_Button4);
// Create and add the button 5
m_Button5 = new JButton("Button 5");
m_Button5.setBorder(BorderFactory.createBevelBorder(BevelBorder.RAISED));
m_Button5.addActionListener(
new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
}
});
// Set the constraints
gbl.setConstraints(m_Button5, gbc);
m_MainPanel.add(m_Button5);
}
}
/* GridBagConstraints
* anchor
* Used when the component is smaller than its display area to determine
* where (within the display area) to place the component. There are two
* kinds of possible values: relative and absolute. Relative values are
* interpreted relative to the container's ComponentOrientation property
* while absolute values are not. Valid values are:
* Absolute Values Relative Values
* GridBagConstraints.NORTH GridBagConstraints.PAGE_START
* GridBagConstraints.SOUTH GridBagConstraints.PAGE_END
* GridBagConstraints.WEST GridBagConstraints.LINE_START
* GridBagConstraints.EAST GridBagConstraints.LINE_END
* GridBagConstraints.NORTHWEST GridBagConstraints.FIRST_LINE_START
* GridBagConstraints.NORTHEAST GridBagConstraints.FIRST_LINE_END
* GridBagConstraints.SOUTHWEST GridBagConstraints.LAST_LINE_START
* GridBagConstraints.SOUTHEAST GridBagConstraints.LAST_LINE_END
* GridBagConstraints.CENTER (the default)
* fill
* Used when the component's display area is larger than the component's
* requested size to determine whether (and how) to resize the component.
* Possible values are GridBagConstraints.NONE (the default),
* GridBagConstraints.HORIZONTAL (make the component wide enough to fill
* its display area horizontally, but don't change its height),
* GridBagConstraints.VERTICAL (make the component tall enough to fill
* its display area vertically, but don't change its width), and
* GridBagConstraints.BOTH (make the component fill its display area
* entirely).
* gridwidth, gridheight
* Specifies the number of cells in a row (for gridwidth) or column
* (for gridheight) in the component's display area. The default value
* is 1. Use GridBagConstraints.REMAINDER to specify that the
* component's display area will be from gridx to the last cell in the
* row (for gridwidth) or from gridy to the last cell in the column
* (for gridheight). Use GridBagConstraints.RELATIVE to specify that
* the component's display area will be from gridx to the next to the
* last cell in its row (for gridwidth or from gridy to the next to the
* last cell in its column (for gridheight).
* insets
* Specifies the component's external padding, the minimum amount of
* space between the component and the edges of its display area.
* ipadx, ipady
* Specifies the component's internal padding within the layout, how much
* to add to the minimum size of the component. The width of the
* component will be at least its minimum width plus ipadx pixels.
* Similarly, the height of the component will be at least the minimum
* height plus ipady pixels.
* gridx, gridy
* Specifies the cell containing the leading corner of the component's
* display area, where the cell at the origin of the grid has address
* gridx = 0, gridy = 0. Use GridBagConstraints.RELATIVE (the default
* value) to specify that the component be placed immediately following
* (along the x axis for gridx or the y axis for gridy) the component
* that was added to the container just before this component was added.
* weightx, weighty
* Used to determine how to distribute space, which is important for
* specifying resizing behavior. Unless you specify a weight for at least
* one component in a row (weightx) and column (weighty), all the components
* clump together in the center of their container. This is because when the
* weight is zero (the default), the GridBagLayout object puts any extra
* space between its grid of cells and the edges of the container.
*/